Getting Started with API Hub for Contract Testing
Tip
API Hub for Contract Testing makes it easy for many developers and testers to test and deploy microservices.
Using the Pact framework, create consumer and provider mocks in-platform or use a range of BYO tools to do so through the Bi-Directional Contract Testing feature.
Prerequisites
You need the following prerequisites to get started with API Hub for Contract Testing:
API Hub subscription for one of the available plans.
API Hub Management
If you want to read more about the API Hub management, visit the following pages:
Note
If you can see the following widgets, you are on the new SmartBear Admin system. Read more about the Trial Subscription here.
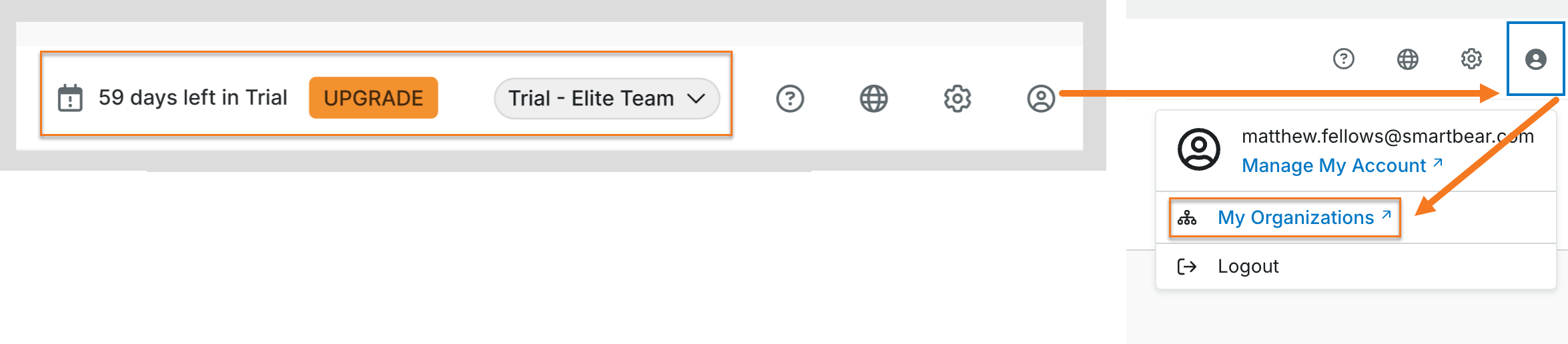
Learn about contract testing
Videos
To learn more about contract testing, watch the introductory-level video series on contract-testing for better understanding.
Workshops
See contract testing in action by completing short and easy in-browser tutorials.
Then, enroll in API Hub for Contract Testing University to learn more about the basics of contract testing through to advanced use cases including a CI/CD integration to help you scale
Essential reading
Here are some key resources to help you on your way:
You can find documentation on all the API Hub for Contract Testing screens under API Hub for Contract Testing User Interface.
Getting help
Here are some ways you can get extra support and have your questions answered:
Pact Slack
We have a Slack workspace where you can chat with other community members and get general support with contract testing or API Hub for Contract Testing. Join us by registering here.
Please ask API Hub for Contract Testing specific questions in one of the #pactflow
channels below (maintained by SmartBear employees), and direct language specific questions to their respective channel such as #pact-jvm
for Java or JVM related questions (maintained by the community).
For API Hub for Contract Testing specific questions, see the following channels:
Getting Started with API Hub for Contract Testing
Join a contract testing and API Hub for Contract Testing expert for a monthly one hour drop-in session to learn the basics of contract testing with API Hub for Contract Testing and have your questions answered. See the next upcoming session and register to attend.
Stack Overflow
Stack Overflow is another place you can search for support, the following link will show all questions tagged with pact
.
SmartBear customer care and solutions engineers
If you need API Hub for Contract Testing specific technical support, contact us or for help with a PoC or plan options get in touch with sales.
Migrating from Pact Broker
When you sign up to the SaaS API Hub for Contract Testing platform, we create a new hosted database for you. However, if you previously used the open-source Pact Broker for your contract testing needs we migrate the data so that nothing is lost. The process involves:
Moving your existing database to the hosted environment associated with your API Hub for Contract Testing account.
Updating it for full compatibility with API Hub for Contract Testing. This requires some scheduled down time for the service, including contract publishing and 'can-i-deploy' webhook.
To get started with migrating from Pact Broker contact us with the subject 'migrating from Pact Broker'.
Note
Applies to SaaS (Hosted) API Hub for Contract Testing accounts only.
If you want to migrate from On-Premises to the SaaS platform, check out our on-prem migration guide here.
Configuring your API token
Note
You cannot use your username and password to access the API Hub for Contract Testing API.
To publish or verify contracts you need to use one of the bearer tokens from the API Tokens section of your API Hub for Contract Testing settings page.
Open your API Hub for Contract Testing account in a browser and log in with your username and password. Click on the settings icon (the cog wheel icon at the top right of the screen).
Then you can see the API Tokens page with two tokens listed - a read-only token and a read/write token.
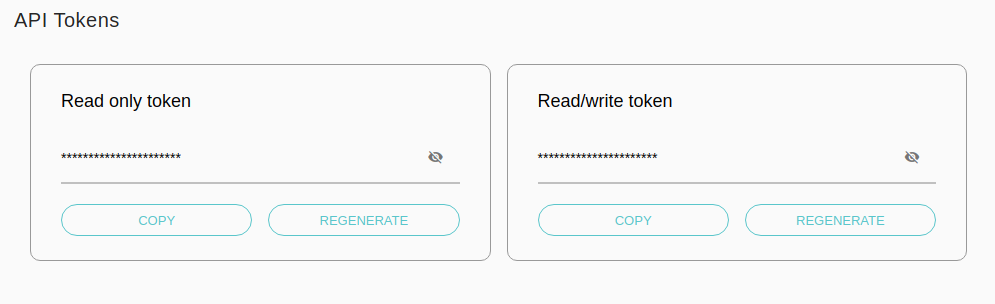
Contracts and verification results are generally only published from a CI machine, so use the read only token on a local development machine and keep the read/write token for CI.
While each of the following examples shows the use of a hardcoded token, note that you would normally be accessing the token via an environment variable or build parameter that is stored and provided in a secure manner (for example, a Jenkins build secret or a Travis encrypted environment variable).
To configure the token, click the tab for your chosen language:
Consumer
Pact JS (Node JS)
Pact-JS documentation for all the pact publication options.
const { Publisher } = require("@pact-foundation/pact") const opts = { pactBroker: 'https://<YOUR_BROKER>.pactflow.io', pactBrokerToken: '<TOKEN>', consumerVersion: process.env.GIT_COMMIT pactFilesOrDirs: ['./pacts'], }; new Publisher(opts).publishPacts()
Java / JUnit 5
Pacj-JVM documentation for all the pact publication options.
pact { publish { providerVersion = { '<GIT_COMMIT>' } //yes, this field name is correct :( pactBrokerUrl = 'https://<YOUR_BROKER>.pactflow.io/' pactBrokerToken = '<TOKEN>' } }
Java / JUnit 4
pact { publish { providerVersion = { '<GIT_COMMIT>' } //yes, this field name is correct :( pactBrokerUrl = 'https://<YOUR_BROKER>.pactflow.io/' pactBrokerToken = '<TOKEN>' } }
Java / Gradle
pact { publish { providerVersion = { '<GIT_COMMIT>' } //yes, this field name is correct :( pactBrokerUrl = 'https://<YOUR_BROKER>.pactflow.io/' pactBrokerToken = '<TOKEN>' } }
Golang
p := dsl.Publisher{} err := p.Publish(types.PublishRequest{ PactURLs: []string{"/path/to/pact/file"}, PactBroker: "https://<YOUR_BROKER>.pactflow.io", ConsumerVersion: "<GIT_COMMIT>", BrokerToken: "<TOKEN>", })
Ruby
Pact Ruby documentation for all the pact publishing options.
require 'pact_broker/client/tasks' PactBroker::Client::PublicationTask.new do | task | task.consumer_version = ENV['GIT_COMMIT'] task.pact_broker_base_url = "https://<YOUR_BROKER>.pactflow.io" task.pact_broker_token = "<TOKEN>" end
.NET
PactNet documentation for all the pact publishing options.
var pactPublisher = new PactPublisher("http://<YOUR_BROKER>.pactflow.io", new PactUriOptions("<TOKEN>")); pactPublisher.PublishToBroker("/pact/to/pacts/dir", Environment.GetEnvironmentVariable("GIT_COMMIT"));
Docker
Pact CLI Docker documentation for all the pact publishing options.
docker run --rm \ -v ${PWD}:${PWD} \ -e PACT_BROKER_BASE_URL="https://<YOUR_BROKER>.pactflow.io" \ -e PACT_BROKER_TOKEN="<TOKEN>" \ pactfoundation/pact-cli:latest \ publish \ ${PWD}/pacts \ --consumer-app-version ${GIT_COMMIT}
Provider
Pact JS (Node JS)
Pact-JS documentation for all the pact verification options.
const { Verifier } = require("@pact-foundation/pact"); return new Verifier().verifyProvider({ provider: "<Your provider name here>", providerBaseUrl: "http://localhost:8081", // Fetch pacts from broker pactBrokerUrl: "https://<YOUR_BROKER>.pactflow.io/", pactBrokerToken: "<TOKEN>", publishVerificationResult: process.env.CI === "true", providerVersion: process.env.GIT_COMMIT, });
Java / JUnit 5
Pact-JVM documentation for all the pact verification options.
@Provider("<Your provider name here>") @PactBroker(host = "<YOUR_BROKER>.pactflow.io", scheme = "https", authentication = @PactBrokerAuth(scheme = "bearer", username = "<TOKEN>", password = "")) public class PactJUnitBrokerTest { @TestTemplate @ExtendWith(PactVerificationInvocationContextProvider.class) void testTemplate(Pact pact, Interaction interaction, HttpRequest request, PactVerificationContext context) { context.verifyInteraction(); } }
Java / JUnit 4
Pact-JVM documentation for all the pact verification options.
@RunWith(PactRunner.class) @Provider("<Your provider name here>") @PactBroker(host = "<YOUR_BROKER>.pactflow.io", scheme = "https", authentication = @PactBrokerAuth(scheme = "bearer", username = "<TOKEN>", password = "")) public class PactJUnitBrokerTest { @TestTarget public final Target target = new HttpTarget(8080); }
Java / Gradle
Pact-JVM documentation for all the pact verification options.
// To turn on the verification publishing, // set the project property `pact.verifier.publishResults` to `true` pact { serviceProviders { '<Your provider name here>' { providerVersion = { '<GIT_COMMIT>' } hasPactsFromPactBroker('https://<YOUR_BROKER>.pactflow.io/', authentication: ['Bearer', '<TOKEN>']) } } }
Golang
_, err := pact.VerifyProvider(t, types.VerifyRequest{ ProviderBaseURL: fmt.Sprintf("http://127.0.0.1:%d", port), BrokerURL: "https://<YOUR_BROKER>.pactflow.io", BrokerToken: "<TOKEN>", PublishVerificationResults: true, ProviderVersion: "<GIT_COMMIT>" })
Ruby
Pact Ruby documentation for all the verification options.
Pact.service_provider "<Your provider name here>" do app_version ENV['GIT_COMMIT'] publish_verification_results ENV['CI'] == 'true' honours_pacts_from_pact_broker do pact_broker_base_url "https://<YOUR_BROKER>.pactflow.io", { token: "<TOKEN>" } end end
.NET
PactNet documentation for all the pact verification options.
var ProviderVersion = Environment.GetEnvironmentVariable("GIT_COMMIT"); var PublishVerificationResults = "true".Equals(Environment.GetEnvironmentVariable("CI")); var config = new PactVerifierConfig { ProviderVersion, //NOTE: Setting a provider version is required for publishing verification results PublishVerificationResults }; IPactVerifier pactVerifier = new PactVerifier(config); pactVerifier .ServiceProvider("<Your provider name here>", "http://your-test-provider-url") .PactBroker("https://<YOUR_BROKER>.pactflow.io", uriOptions: new PactUriOptions("<TOKEN>")) .Verify();
Docker
version: "3" services: api: image: "your image" expose: - "9292" pact_verifier: image: pactfoundation/pact-cli:latest depends_on: - api environment: - PACT_BROKER_BASE_URL="https://<YOUR_BROKER>.pactflow.io" - PACT_BROKER_TOKEN="<TOKEN>" command: > verify --provider-base-url http://api:9292 --provider "<Your provider name here>"
To run
docker-compose -f docker-compose-verify.yml up \ --build --abort-on-container-exit --exit-code-from pact_verifier
Supported Tools & Languages
Pact is available in many different implementations. See the table here.